Image resizing is the process of changing the dimensions of an image while preserving its aspect ratio. It is a common preprocessing step in computer vision applications, including object detection, image classification, and image segmentation.
The primary reasons for resizing images are as follows:
- To fit the image into a specific display size or aspect ratio, such as for web pages or mobile applications.
- To reduce the computational complexity of processing the image, such as for real-time computer vision applications or when the image size is too large to fit into memory.
- When resizing an image, we need to decide on a new size for the image. The new size can be specified in terms of pixels or as a scaling factor. In the latter case, we multiply the original image dimensions by a scaling factor to obtain the new dimensions.
There are two primary methods for resizing an image: interpolation and resampling:
Interpolation is a technique for estimating the pixel values in the resized image. It involves computing a weighted average of the pixel values in the original image surrounding the target pixel location. There are several interpolation methods available, including nearest neighbor, bilinear, bicubic, and Lanczos resampling.
Lanczos resampling is a method used in digital image processing for resizing or resampling images. It is a type of interpolation algorithm that aims to produce high-quality results, particularly when downscaling images. The Lanczos algorithm is named after Cornelius Lanczos, a Hungarian mathematician and physicist. The Lanczos resampling algorithm involves applying a sinc function (a type of mathematical function) to the pixel values in the original image to calculate the values of pixels in the resized image. This process is more complex than simple interpolation methods such as bilinear or bicubic, but it tends to produce better results, especially when reducing the size of an image.
The following is a simple example in Python using the Pillow library (a fork of PIL) to demonstrate nearest neighbor, bilinear, bicubic, and Lanczos resampling methods:
from PIL import Image
# Open an example image
image_path = “../images/roseflower.jpeg”
image = Image.open(image_path)
# Resize the image using different interpolation methods
nearest_neighbor_resized = image.resize((100, 100), \
resample=Image.NEAREST)
bilinear_resized = image.resize((100, 100), \
resample=Image.BILINEAR)
bicubic_resized = image.resize((100, 100), \
resample=Image.BICUBIC)
lanczos_resized = image.resize((100, 100), \
resample=Image.LANCZOS)
# Save the resized images
nearest_neighbor_resized.save(“nearest_neighbor_resized.jpg”)
bilinear_resized.save(“bilinear_resized.jpg”)
bicubic_resized.save(“bicubic_resized.jpg”)
lanczos_resized.save(“lanczos_resized.jpg”)
We get the following output:
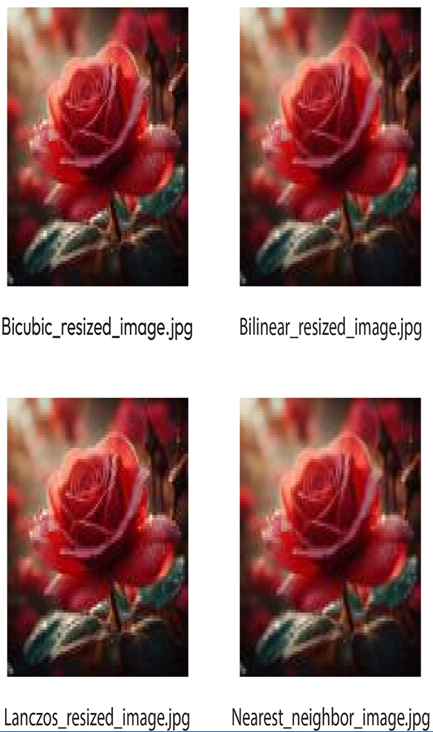
Figure 4.9 – The results of each interpolation method
Let’s delve into the details of each interpolation method:
- Nearest neighbor method (Image.NEAREST): This method chooses the nearest pixel value to the interpolated point:
- Usage (resample=Image.NEAREST): Simple and fast. Often used for upscaling pixel art images.
- Visual effect: Results in blocky or pixelated images, especially noticeable during upscaling.
- Bilinear method (Image.BILINEAR): Uses a linear interpolation between the four nearest pixels:
- Usage (resample=Image.BILINEAR): Commonly used for general image resizing
- Visual effect: Smoother than nearest neighbor but may result in some loss of sharpness
- Bicubic method (Image.BICUBIC): Employs a cubic polynomial for interpolation:
- Usage (resample=Image.BICUBIC): Typically used for high-quality downsampling
- Visual effect: Smoother than bilinear; often used for photographic images, but can introduce slight blurring
- Lanczos method (Image.LANCZOS): Applies a sinc function as the interpolation kernel:
- Usage (resample=Image.LANCZOS): Preferred for downscaling images and maintaining quality.
- Visual effect: Generally produces the highest quality, especially noticeable in downscaling scenarios. May take longer to compute.
- Choosing the right method:
- Quality versus speed: Nearest neighbor is the fastest but may result in visible artifacts. Bicubic and Lanczos are often preferred for quality, sacrificing a bit of speed.
- Downscaling versus upscaling: Bicubic and Lanczos are commonly used for downscaling, while bilinear might be sufficient for upscaling.