Let us see some example Python code for image augmentation. We first import the necessary libraries: keras.preprocessing.image for image augmentation and os for file and directory operations:
Step 1: Import the necessary libraries for image augmentation
The following code snippet shows how to import the libraries:
# import the necessary libraries
from keras.preprocessing.image import ImageDataGenerator
import os
We define the path to the image directory as follows:
# Define the path to the image directory
img_dir = ‘path/to/image/directory’
Step 2: Create an instance of ImageDataGenerator
We create an instance of the ImageDataGenerator class, which allows us to define various types of image augmentation techniques. In this example, we use rotation, horizontal and vertical shifts, shear, zoom, and horizontal flipping:
# Create an instance of the ImageDataGenerator class
datagen = ImageDataGenerator(
rotation_range=30,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode=’nearest’)
Step 3: Load each image from the directory and convert the image to an array
We get a list of all image filenames in the directory using a list comprehension. We then loop through all the image files using a for loop. For each image file, we load the image using Keras’ load_img function and convert it to an array using Keras’ img_to_array function:
# Get a list of all image filenames in the directory
img_files = [os.path.join(img_dir, f) \
for f in os.listdir(img_dir) \
if os.path.isfile(os.path.join(img_dir, f))]
# Loop through all the image files
for img_file in img_files:
# Load the image using Keras’ load_img function
img = load_img(img_file)
# Convert the image to an array using Keras’ img_to_array function
img_arr = img_to_array(img)
We reshape the array to have a batch dimension of 1, which is required by the flow method of the ImageDataGenerator class:
# Reshape the array to have a batch dimension of 1
img_arr = img_arr.reshape((1,) + img_arr.shape)
Step 4: Regenerate five augmented images for each input image
We then regenerate our augmented images as follows:
# Generate 5 augmented images for each input image
i = 0
for batch in datagen.flow( \
img_arr, batch_size=1, save_to_dir=img_dir, \
save_prefix=’aug_’, save_format=’jpg’ \
):
i += 1
if i == 5:
break
You can see five augmented images generated for the flow in the GitHub repository in the directory as follows:
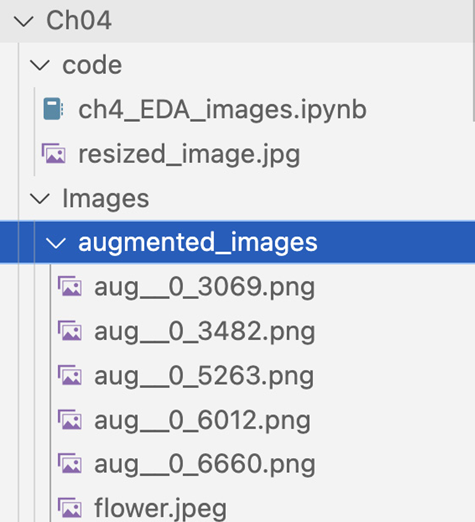
Figure 4.12 – Augmented images
We use the flow method to generate five augmented images for each input image. The flow method takes the array of input images, a batch size of 1, and various parameters defined in step 3. It returns a generator that generates augmented images on the fly. We save each augmented image with a filename prefix of aug_ using the save_to_dir, save_prefix, and save_format parameters.
In this section, we learned how to transform a dataset using data augmentation and saw some commonly used data augmentation techniques for generating additional data for training.
Summary
In this chapter, we learned how to review images after loading an image dataset and explore them using a tool called Matplotlib in Python. We also found out how to change the size of pictures using two handy tools called PIL and OpenCV. And just when things were getting interesting, we discovered a cool trick called data augmentation that helps us make our dataset bigger and teaches our computer how to understand different versions of the same picture.
But wait, there’s more to come! In the next chapter, we are going to see how to label our image data using Snorkel based on rules and heuristics. Get ready for some more fun as we dive into the world of labeling images!